Wolt Drive API webhook service
Webhooks provide a mechanism for the merchant to receive information related to e.g. status changes of a single delivery. The Wolt Drive API contains CRUD endpoints for managing the webhooks.
The webhooks operate in the merchant level: the webhook events related to all the venues of the merchant will be sent to the same endpoint. I.e. the merchant needs to configure only a single webhook in order to receive all the events related to all the stores.
The merchant system will need to provide a callback_url
and client_secret
for each webhook it registers.
Each event is encoded into a JSON Web Token (JWT) and will be sent in the format: "token": "<payload as HS256 encoded JWT>"
. After receiving and decoding the payload, the system can validate the event origin by verifying the secret (signature) which it provided while registering the webhook. Signature is not base64 encoded.
The following sequence diagram visualizes the webhook related operations.
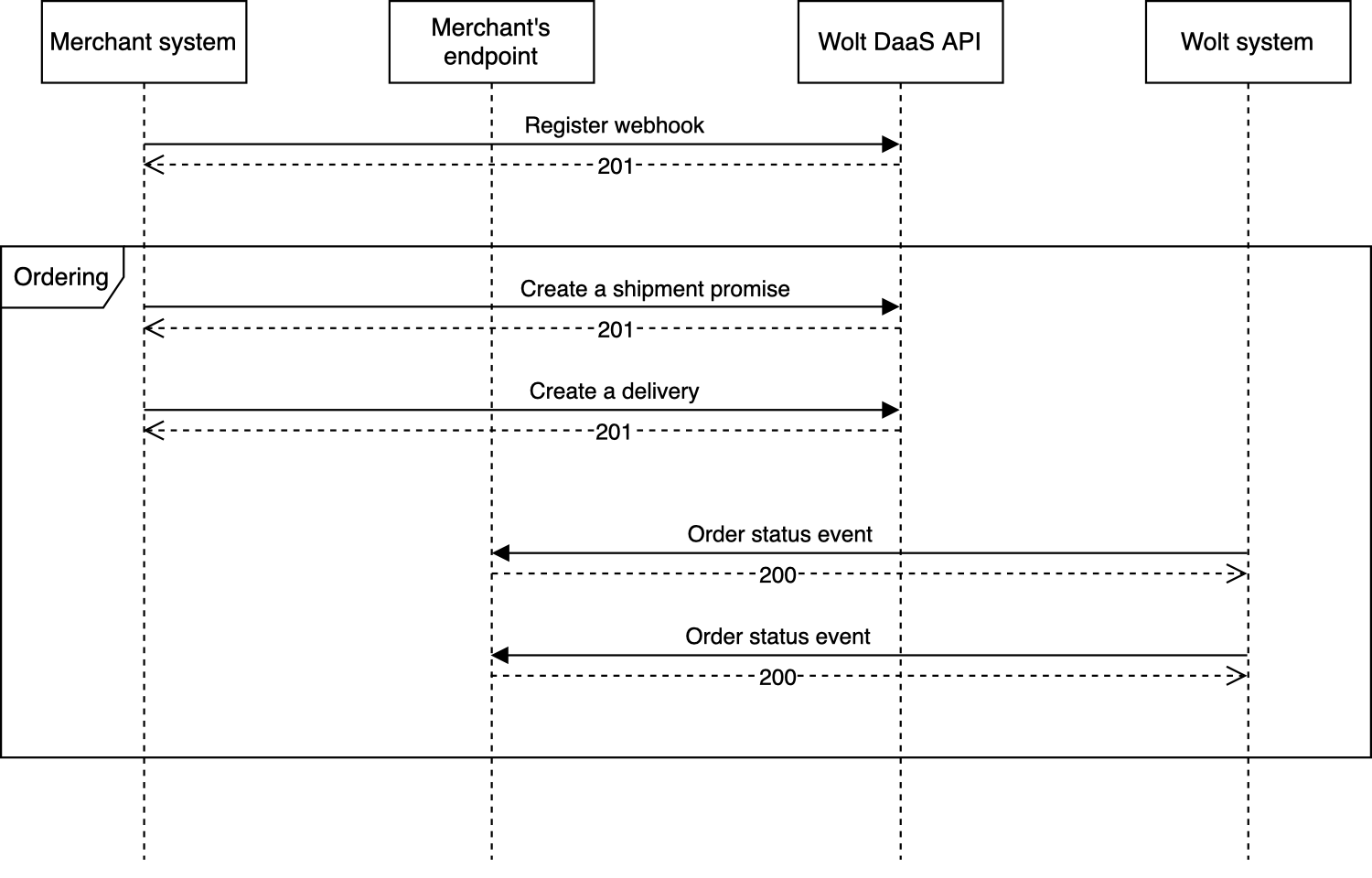
Endpoints for managing webhooks
All requests to Drive API endpoints must be authorized with a Bearer Token.
All the requests must contain Authorization: Bearer <token>
header. This token is often referred as Merchant Key. Staging token will be provided by Wolt when you start the integration development and the production token when you successfully complete the testing phase. Only one token per merchant is needed.
Create a webhook
Create a webhook to be used to send events
POST /v1/merchants/{merchant_id}/webhooks
POST /v1/merchants/{merchant_id}/webhooks
{"callback_config": {"exponential_retry_backoff": {"exponent_base": 2,"max_retry_count": 10}},"callback_url": "string","client_secret": "string","disabled": false}
Name | In | Type | Required | Description |
---|---|---|---|---|
merchant_id | path | string | true | |
body | body | WebhookCreatePayloadV1 | true |
Create webhook response
201 Created{"callback_config": {"exponential_retry_backoff": {"exponent_base": 0,"max_retry_count": 0}},"callback_url": "string","created_at": "string","disabled": true,"id": "string","modified_at": "string","subscribed_events": ["string"]}
Status | Meaning | Description |
---|---|---|
201 | Created | Successful Response |
400 | Bad Request | Bad Request |
401 | Unauthorized | Unauthorized |
422 | Unprocessable Entity | Validation Error |
Get a list of webhooks
Get a list of all webhook configured under the merchant ID.
GET /v1/merchants/{merchant_id}/webhooks
GET /v1/merchants/{merchant_id}/webhooks
curl {{host}}/v1/merchants/{merchant_id}/webhooks \-H "Authorization: Bearer (token)" \-H "Content-Type: application/json"
Name | In | Type | Required |
---|---|---|---|
merchant_id | path | string | true |
List all webhooks response
200 Ok[{"callback_config": {"exponential_retry_backoff": {"exponent_base": 0,"max_retry_count": 0}},"callback_url": "string","created_at": "string","disabled": true,"id": "string","modified_at": "string","subscribed_events": ["string"]}]
Parameter | Type | Required | Description |
---|---|---|---|
callback_config | WebhookCallbackConfigV1 | Required | |
callback_url | string | Required | End-point url where webhook events are sent. |
created_at | string | Required | |
disabled | boolean | Required | Determines whether the webhook is in use and events should be sent. |
id | string | Required | |
modified_at | string | Required | |
subscribed_events | [string] | Required | The list of events a webhook sends updates about. See available events |
Status | Meaning | Description |
---|---|---|
200 | Ok | Successful Response |
400 | Bad Request | Bad Request |
401 | Unauthorized | Unauthorized |
422 | Unprocessable Entity | Validation Error |
Get a single webhook
GET /v1/merchants/{merchant_id}/webhooks/{webhook_id}
GET /v1/merchants/{merchant_id}/webhooks/{webhook_id}
curl {{host}}/v1/merchants/{merchant_id}/webhooks/{webhook_id} \-H "Authorization: Bearer (token)" \-H "Content-Type: application/json"
Get webhook request parameters
Name | Location | Type | Required |
---|---|---|---|
merchant_id | In: path | string | Required |
webhook_id | In: path | string | Required |
Get a webhooks response
200 Ok{"callback_config": {"exponential_retry_backoff": {"exponent_base": 0,"max_retry_count": 0}},"callback_url": "string","created_at": "string","disabled": true,"id": "string","modified_at": "string","subscribed_events": ["string"]}
Parameter | Type | Required | Description |
---|---|---|---|
callback_config | WebhookCallbackConfigV1 | Required | |
callback_url | string | Required | End-point url where webhook events are sent. |
created_at | string | Required | |
disabled | boolean | Required | Determines whether the webhook is in use and events should be sent. |
id | string | Required | |
modified_at | string | Required | |
subscribed_events | [string] | Required | The list of events a webhook sends updates about. See available events |
Status | Meaning | Description |
---|---|---|
200 | Ok | Successful Response |
400 | Bad Request | Bad Request |
401 | Unauthorized | Unauthorized |
422 | Unprocessable Entity | Validation Error |
Get webhook response
200 Ok{"callback_config": {"exponential_retry_backoff": {"exponent_base": 0,"max_retry_count": 0}},"callback_url": "string","created_at": "string","disabled": true,"id": "string","modified_at": "string","subscribed_events": ["string"]}
Update a webhook
Change the webhook configuration and the subscribed events.
PATCH /v1/merchants/{merchant_id}/webhooks/{webhook_id}
PATCH /v1/merchants/{merchant_id}/webhooks/{webhook_id}
{"callback_config": {"exponential_retry_backoff": {"exponent_base": 0,"max_retry_count": 0}},"callback_url": "string","client_secret": "string","disabled": false,"subscribed_events": ["order.received"]}
Name | In | Type | Required | Description |
---|---|---|---|---|
webhook_id | path | string | true | |
merchant_id | path | string | true | |
body | body | WebhookUpdatePayload | true |
200 Ok{"callback_config": {"exponential_retry_backoff": {"exponent_base": 0,"max_retry_count": 0}},"callback_url": "string","created_at": "string","disabled": true,"id": "string","modified_at": "string","subscribed_events": ["string"]}
Delete a webhook
DELETE /v1/merchants/{merchant_id}/webhooks/{webhook_id}
DELETE /v1/merchants/{merchant_id}/webhooks/{webhook_id}
curl -X DELETE {{host}}/v1/merchants/{merchant_id}/webhooks/{webhook_id} \-H "Authorization: Bearer (token)" \-H "Content-Type: application/json"
204 No content
Webhook order events
Note that we release new features constantly and may add optional fields in our API without creating a new API version. In practice, this could mean that some new fields are also introduced in the webhook payloads. The client side should allow unknown events and keys in the payloads.
Events that are triggered by the same action, like order.dropoff_completed
and order.delivered
, may be sent at seemingly random order.
Webhook order event types
Event type | Auto-subscribed | Description |
---|---|---|
order.received | Yes | Order has been received successfully. |
order.rejected | Yes | Order has been rejected. |
order.pickup_eta_updated | Yes | Order pickup ETA is updated. New time is included in event details. |
order.pickup_started | Yes | Courier has started a pickup task. |
order.picked_up | Yes | Order has been picked up from venue. |
order.pickup_arrival | Yes | Courier is arriving to pickup location. Triggered when courier enters 150 meter geo-fence radius. |
order.dropoff_started | Yes | Courier has started a dropoff task. |
order.dropoff_arrival | Yes | Courier is arriving to dropoff location. Triggered when courier enters 150 meter geo-fence radius. |
order.dropoff_completed | Yes | Courier has completed the dropoff task. |
order.delivered | Yes | Complete order has been delivered. |
order.customer_no_show | No | Customer not reached and delivery could not be made. |
order.location_updated | No | Courier location info. When enabled, expect heavy event load and consider using a separate configuration for this event. |
order.dropoff_eta_updated | Yes | Order dropoff ETA is updated. |
order.handshake_delivery | Yes | Sent when delivery handshake verification is enabled. |
Order event payload
Example payload format does not apply to order.location_updated
and order.handshake_delivery
events. Notice that some fields may be null
in some events. Event details refer to order details.
The real payload format of all webhook events is {"token": "<payload as HS256 encoded JWT>"}
. The schemas documented here for the body refer to the decoded payload.
{"dispatched_at": "2023-01-01T00:00:00.000Z","type": "order.received","details": {"id": "string","venue_id": "string","wolt_order_reference_id": "string","tracking_reference": "string","merchant_order_reference_id": "string","order_number": "string","price": {"amount": 0,"currency": "string"},"pickup": {"eta": "2023-01-01T00:00:00.000Z"},"dropoff": null,"courier": null,"parcels": []}}
Parameter | Type | Required | Description |
---|---|---|---|
dispatched_at | string | Required | Timestamp is in ISO8601 format e.g. 2020-01-30T10:00:13.123Z |
type | OrderEventType | Required | An enumeration. |
id | string | Required | Event ID. |
venue_id | string | Required | Wolt venue ID. |
wolt_order_reference_id | string | Required | Wolt order reference ID. |
tracking_reference | string | Required | Wolt tracking reference. |
merchant_order_reference_id | string | The merchant_order_reference_ID received in delivery creation. | |
order_number | string | The order_number received in delivery creation. | |
price | Price | Required | Price for delivery. |
pickup.eta | string | Required | Pickup ETA. Timestamp is in ISO8601 format e.g. 2020-01-30T10:00:13.123Z |
dropoff | WebhookDropoffEta | Required | Included only in order.dropoff_eta_updated and order.delivered events. Otherwise null. |
courier | Courier | Required | Info on assigned courier. Included only in order.pickup_started event. Otherwise null. |
parcels | WebhookParcelArray | Required | Array of related parcel items. Included only in order.picked_up and order.dropoff_completed events. Otherwise null. |
Location updated
This event type order.location_updated
is optional. When enabled, expect a heavy event load and consider creating a separate configuration for the event. Location refers to the current location of assigned courier partner.
{"dispatched_at": "2023-01-01T00:00:00.000Z","type": "order.location_updated","details": {"id": "string","wolt_order_reference_id": "string","merchant_order_reference_id": "string","order_number": "string","tracking_id": "string","courier_location": {"lat": 0.0,"lon": 0.0}}}
Parameter | Type | Required | Description |
---|---|---|---|
dispatched_at | string | Required | Timestamp is in ISO8601 format e.g. 2020-01-30T10:00:13.123Z |
type | OrderEventType | Required | An enumeration. |
id | string | Required | Event ID. |
wolt_order_reference_id | string | Required | Wolt order reference ID. |
merchant_order_reference_id | string | The merchant_order_reference_ID received in delivery creation. | |
order_number | string | The order_number received in delivery creation. | |
tracking_id | string | Required | Courier tracking ID. |
courier_location.lat | number | Required | Latitude value in EPSG:3857 Coordinate Reference System |
courier_location.lon | number | Required | Latitude value in EPSG:3857 Coordinate Reference System |
Handshake delivery
This event type order.handshake_delivery
is only sent when the order has delivery handshake verification enabled.
{"dispatched_at": "2023-01-01T00:00:00.000Z","type": "order.handshake_delivery","details": {"id": "string","wolt_order_reference_id": "string","merchant_order_reference_id": null,"order_number": "string","pin_code": "string"}}
Parameter | Type | Required | Description |
---|---|---|---|
dispatched_at | string | Required | Timestamp is in ISO8601 format e.g. 2020-01-30T10:00:13.123Z |
type | OrderEventType | Required | An enumeration. |
id | string | Required | Event ID. |
wolt_order_reference_id | string | Required | Wolt order reference ID. |
merchant_order_reference_id | string | The merchant_order_reference_ID received in delivery creation. | |
order_number | string | The order_number received in delivery creation. | |
pin_code | string | Required | The PIN code assigned for the delivery. |